Motor:
Unipolar stepper motor


Bipolar Stepper Motor

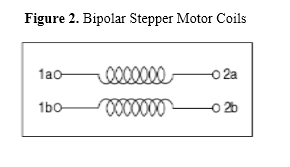

Step Angle
Driving Unipolar Stepper
Full Step
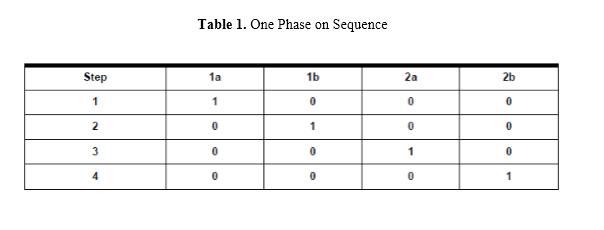
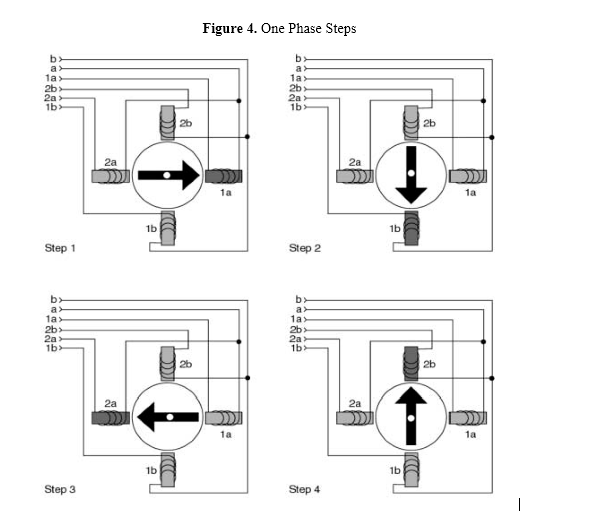

Two Phases on Mode



Half Step Mode

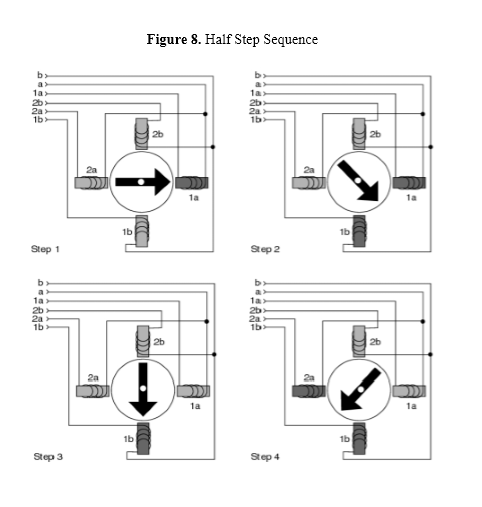


Micro step
Driving Bipolar Stepper

Advantages
Applications
/* Name : unipolar_FS.c * Purpose : Source code for Unipolar Fullstep Stepper Motor with PIC18F4550. * Author : Gemicates * Date : 2017-06-29 * Website : www.gemicates.org * Revision : None */ /**** Full step drive Mode****/ #include <htc.h> // Header file for PIC18F4550 series #define _XTAL_FREQ 12000000 // 12MHz Crystal Frequency for PIC18F4550 void delay_ms(); // delay declaration unsigned int i; void main() // main fuction { PORTD = 0; TRISD = 0; // Configure PORTD as output while(1) { // loop executed infinite times for(i=0;i<49;i++) { PORTD=0x09; // 1001 __delay_ms(60); // delay } for(i=0;i<49;i++) { PORTD=0x0C; //1100 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x06; //0110 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x03; //0011 __delay_ms(60); } } }
/* Name : unipolar_HS.c * Purpose : Source code for Unipolar Halfstep Stepper Motor with PIC18F4550. * Author : Gemicates * Date : 2017-06-29 * Website : www.gemicates.org * Revision : None */ /**** Half Drive Stepping Mode ****/ #include <htc.h> // Header file for PIC18F4550 series #define _XTAL_FREQ 12000000 // 12MHz Crystal Frequency for PIC18F4550 void delay_ms(); // delay declaration unsigned int i; void main() // main fuction { PORTD = 0; TRISD = 0; // Configure PORTD as output while(1) { // loop executed infinite times for(i=0;i<49;i++) { PORTD=0x08; // 1000 __delay_ms(60); // delay } for(i=0;i<49;i++) { PORTD=0x0C; // 1100 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x04; // 0100 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x06; // 0110 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x02; // 0010 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x03; // 0011 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x01; // 0001 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x09; // 1001 __delay_ms(60); } } }
/* Name : unipolar_FS.c * Purpose : Source code for Unipolar wave drive Stepper Motor with PIC18F4550. * Author : Gemicates * Date : 2017-06-29 * Website : www.gemicates.org * Revision : None */ /**** Wave Drive Stepping Mode****/ #include <htc.h> // Header file for PIC18F4550 series #define _XTAL_FREQ 12000000 // 12MHz Crystal Frequency for PIC18F4550 void delay_ms(); // delay declaration unsigned int i; void main() // main fuction { PORTD = 0; TRISD = 0; // Configure PORTD as output while(1) { // loop executed infinite times for(i=0;i<49;i++) { PORTD=0x08; // 1000 __delay_ms(60); // delay } for(i=0;i<49;i++) { PORTD=0x04; //0100 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x02; //0010 __delay_ms(60); } for(i=0;i<49;i++) { PORTD=0x01; //0001 __delay_ms(60); } } }
/* Name : bipolar.c * Purpose : Source code for Bipolar Stepper Motor with PIC18F4550. * Author : Gemicates * Date : 2017-06-28 * Website : www.gemicates.org * Revision : None */ /**** Program to interface Bipolar Stepper Motor with PIC18F4550 ****/ #include <htc.h> // Header file for PIC18F4550 series #define _XTAL_FREQ 12000000 // 12MHz Crystal Frequency for PIC18F4550 void delay_ms(); // delay declaration unsigned int i; void main() // main fuction { TRISD = 0b0000000; // PORT D as output port PORTD = 0b0000000; while(1) { // loop executed infinite times for(i=0;i<49;i++) { PORTD= 0b00000001; // 0001 __delay_us(60); // delay } for(i=0;i<49;i++) { PORTD= 0b00000100; //0100 __delay_ms(60); } for(i=0;i<49;i++) { PORTD = 0b00000010; //0010 __delay_ms(60); } for(i=0;i<49;i++) { PORTD = 0b00001000; //1000 __delay_ms(60); } } }